Integrating DecisionRules with Azure Functions: A Step-by-Step Guide
Azure Functions provide a serverless compute platform that allows you to run code without managing infrastructure. In this guide, we'll walk through creating an Azure Function that integrates with DecisionRules, enabling you to execute your business rules through a serverless API.
.webp)
.webp)
Setting Up Your Azure Function
To get started, you'll need Visual Studio Code with the Azure Functions extension installed. Here's how to create and deploy your function:
- Create a new Azure Function project in VS Code using the command palette (Ctrl+Shift+P):
- Select "Azure Functions: Create New Project"
- Choose JavaScript as your language
- Select HTTP trigger as your template
- Name your function (e.g., "decisionRules")
- Set authorization level to "Function"
- Once your project is created, you'll need to install the required dependencies. Open your terminal and run:
npm install @azure/functions axios
The Integration Code
Your Azure Function will act as a middleware between your application and DecisionRules. The function accepts POST requests with your rule ID and input data, then forwards them to DecisionRules' API. Here's what your code should look like:
const { app } = require('@azure/functions');
const axios = require('axios');
app.http('decisionRules', {
methods: ['POST'],
authLevel: 'function',
handler: async (request, context) => {
context.log('DecisionRules function processing request.');
try {
const body = await request.json();
// Validate required fields
if (!body || !body.ruleId) {
return {
status: 400,
jsonBody: {
error: 'Rule ID is required'
}
};
}
// Get API key from environment variables
const apiKey = process.env.DECISIONRULES_API_KEY;
if (!apiKey) {
context.log.error('DecisionRules API key not configured');
return {
status: 500,
jsonBody: {
error: 'API key configuration missing'
}
};
}
// Prepare the request to DecisionRules
const decisionRulesRequest = {
method: 'POST',
url: `https://api.decisionrules.io/rule/solve/${body.ruleId}`,
headers: {
'Authorization': `Bearer ${apiKey}`,
'Content-Type': 'application/json'
},
data: body.data || {}
};
// Add optional version if provided
if (body.version) {
decisionRulesRequest.headers['X-Version'] = body.version;
}
// Call DecisionRules API
const response = await axios(decisionRulesRequest);
// Return successful response
return {
status: 200,
jsonBody: response.data
};
} catch (error) {
context.log.error('Error executing decision rule:', error);
// Handle different types of errors
if (error.response) {
// DecisionRules API error
return {
status: error.response.status,
jsonBody: {
error: 'DecisionRules API error',
details: error.response.data
}
};
}
// Generic error response
return {
status: 500,
jsonBody: {
error: 'Internal server error',
message: error.message
}
};
}
}
});
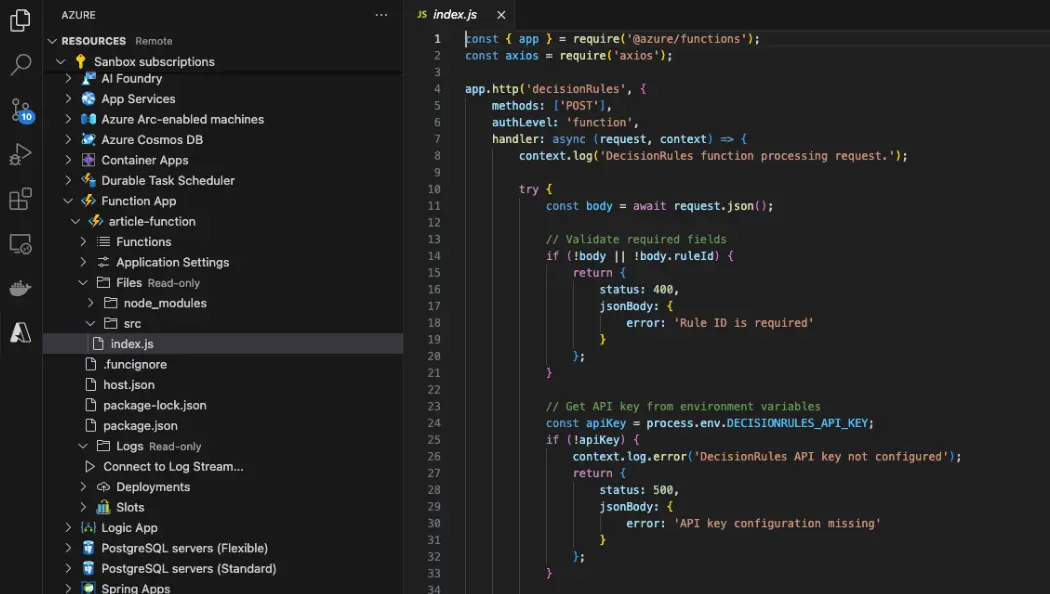
Configuration and Deployment
The most crucial part is securing your DecisionRules API key. Add it as an environment variable in your Azure Function's configuration:
- In Azure Portal, navigate to your Function App
- Go to Settings > Environment variables
- Add a new application setting:
- Name: DECISIONRULES_API_KEY
- Value: Your DecisionRules API key

Using Your Function
Once deployed, your function accepts POST requests with this structure:
{
"ruleId": "your-rule-id",
"data": {
// Your input data for the decision rule
}
}
The function handles:
- Authentication with DecisionRules
- Error handling and validation
- Response formatting
Security Considerations
The function uses Function-level authentication, meaning each request needs an access key. This key is automatically included in your function's URL. Make sure to:
- Keep your function URL and key secure
- Store your DecisionRules API key in environment variables
- Use HTTPS for all requests
This serverless approach offers several benefits:
- Pay-per-execution pricing
- Automatic scaling
- No infrastructure management
- Global deployment options
With this setup, you can now execute your DecisionRules business rules through a serverless API, making it easy to integrate with other applications and services in your ecosystem.